Introduction to TypeScript typeof
Have you ever found yourself struggling to understand the intricacies of TypeScript’s typeof operator? If so, you’re not alone. Many developers find this feature to be a bit confusing at first glance. But fear not! In this comprehensive guide, we will unravel the mysteries of TypeScript typeof and break it down into easy-to-understand concepts. By the end of this article, you’ll have a solid understanding of how typeof works and how it can benefit your TypeScript projects. So grab your favorite beverage, sit back, and let’s dive into the world of TypeScript typeof together!
Why is typeof important in TypeScript?
TypeScript, being a statically typed superset of JavaScript, provides the typeof operator to infer and manipulate types at runtime. This feature plays a crucial role in ensuring type safety and enhancing code understanding and maintainability.
One key benefit of using typeof is that it allows developers to dynamically check the type of a variable or expression. By utilizing this operator, you can write conditional statements that execute different blocks of code based on the detected type. This enables you to handle situations where the behavior may vary depending on whether an object is an array, string, number, or another data type.
Moreover, typeof helps with enforcing stricter compile-time checks by allowing you to validate if a variable or property has been properly initialized before accessing its properties or methods. It empowers developers to catch potential errors early in development rather than encountering them at runtime.
Not only does typeof enable better error handling and prevention but it also improves code readability and documentation. When used correctly, it provides valuable information about variables’ expected types throughout your codebase without resorting to extensive comments.
Additionally, using typeof can be particularly useful when working with external libraries or APIs whose return types may not be explicitly defined within your TypeScript project. By employing this operator along with conditional logic, you can ensure that your application handles any unexpected data appropriately instead of crashing unexpectedly.
In conclusion,
typeof is an essential tool for TypeScript developers as it assists in performing runtime type inference and manipulation while providing improved error handling capabilities. Its usage enhances both code quality and maintainability by enabling dynamic typing checks and facilitating more robust code documentation practices.
Keep these tips in mind as we explore the basic syntax and usage of typeof next!
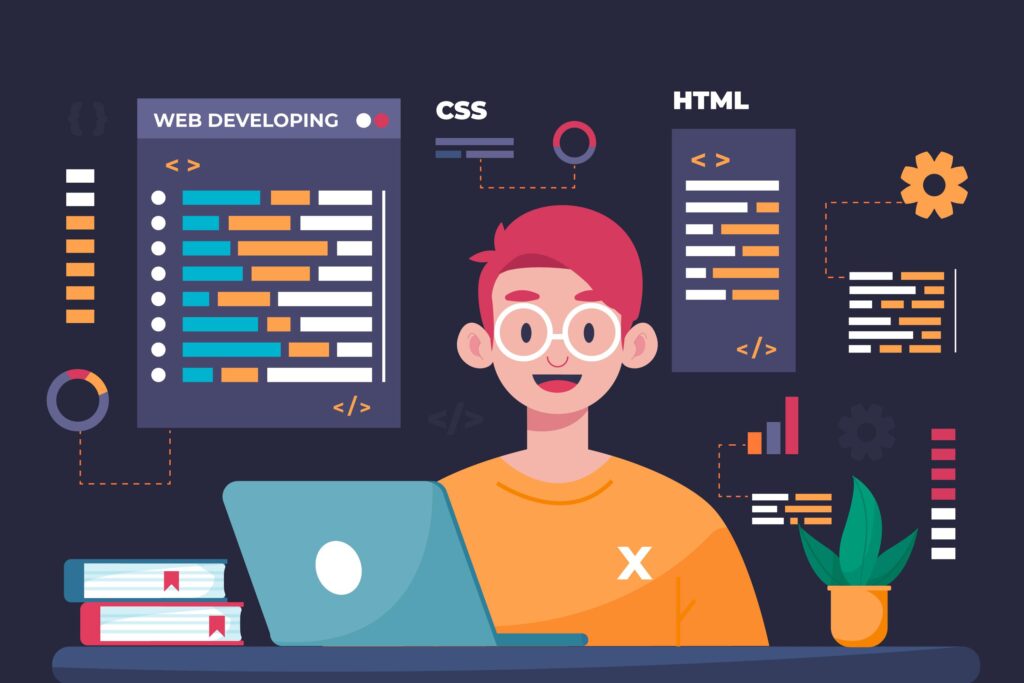
Basic Syntax and Usage of typeof
The basic syntax and usage of typeof in TypeScript is quite straightforward. It allows you to determine the type of a variable or value at runtime. To use typeof, simply precede the variable or value with the keyword “typeof” followed by an expression.
For example, let’s say we have a variable called “age” which holds an integer value. We can use typeof to check its type like this:
“`typescript
let age = 25;
console.log(typeof age); // Output: number
“`
In this case, typeof returns “number” because that’s the data type of the “age” variable.
You can also use typeof on other types such as strings, booleans, objects, arrays, and functions. It works similarly for all these types.
Another useful application of typeof is checking if a variable exists before using it. For instance:
“`typescript
if (typeof name !== ‘undefined’) {
console.log(“Name:”, name);
} else {
console.log(“Name not defined”);
}
“`
This code snippet checks if the variable “name” has been defined before logging its value. If it hasn’t been defined yet, it will print “Name not defined”.
Understanding the basic syntax and usage of typeof in TypeScript is crucial for effectively working with different data types and ensuring your code behaves as expected at runtime without any unexpected errors or bugs.
Common Misconceptions about typeof
When it comes to using the typeof operator in TypeScript, there are a few common misconceptions that developers often have. Let’s take a closer look at these misconceptions and clear up any confusion.
One misconception is that typeof can be used to determine if a variable is null or undefined. However, this is not the case. The typeof operator will return “object” for both null and objects of type object. To check if a variable is null or undefined, you should use the !== operator instead.
Another misconception is that typeof can be used to differentiate between different types of objects. While typeof can distinguish between primitive types such as string, number, boolean, etc., it cannot differentiate between custom objects or arrays. In such cases, you may need to use other techniques like instanceof or checking specific properties.
Some developers also believe that typeof can determine the exact sub-type of an object. For example, they might think that typeof could tell them whether an object is an instance of a specific class or interface.
However, this is not accurate as typeof only returns basic JavaScript types and does not provide information about custom classes or interfaces.
It’s important to understand these common misconceptions so that you don’t rely on incorrect assumptions when using the typeof operator in your TypeScript code. By having a clear understanding of its limitations and capabilities, you can avoid potential pitfalls and make more informed decisions when working with TypeScript.
Remember to always test your code thoroughly and consider alternative approaches when needed to ensure accurate results in your development projects!
Advanced Usage of typescript typeof
When it comes to TypeScript, the typeof operator has more tricks up its sleeve than you might think. It not only allows you to check the type of a variable but also enables some powerful advanced features.
One such feature is using typeof in conditional statements. By combining typeof with logical operators like && and ||, you can create complex conditions based on variable types. This can be especially useful when handling different scenarios based on specific data types.
Another advanced usage of typeof is for type guards. Type guards are a way to narrow down the type of a variable within a certain code block. By using an if statement with the condition `typeof myVariable === ‘string’`, for example, you can ensure that your code will only execute if the variable is indeed a string.
Furthermore, typeof can be used in conjunction with keyof to get all possible keys of an object’s properties. This dynamic approach allows for more flexibility and reduces hardcoding errors.
By understanding these advanced use cases of typeof, you’ll have more control over your code and make it more robust and flexible. So don’t underestimate this seemingly simple operator – explore its capabilities and level up your TypeScript skills!
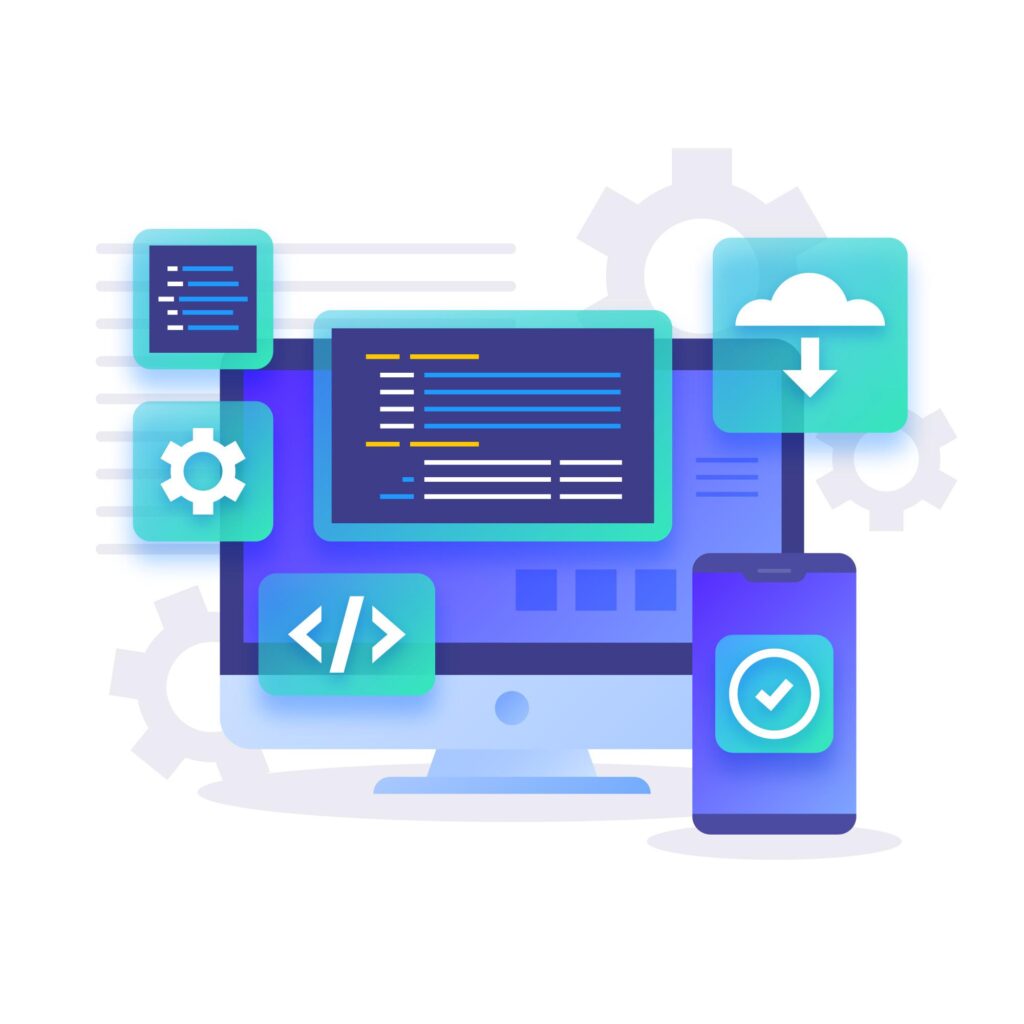
Implementing typeof in Real-World Scenarios
Implementing typeof in Real-World Scenarios
When working with TypeScript, the typeof operator can be incredibly useful in real-world scenarios. It allows you to determine the type of a variable or value at runtime, providing valuable information that can help you make decisions and perform certain actions.
One common scenario where typeof comes in handy is when validating user input. Let’s say you have a form where users enter their age. Using typeof, you can easily check if the entered value is indeed a number before performing any calculations or storing it in your database.
Another practical use case for typeof is when dealing with external APIs. Suppose you’re fetching data from an API that returns different types of objects based on certain conditions. By using typeof, you can dynamically handle and process each response differently based on its type.
Typeof also plays a crucial role when working with libraries and frameworks that rely heavily on dynamic typing. For example, if you’re using React.js and need to conditionally render components based on their type, typeof provides an elegant solution by allowing you to check the component’s type before rendering it.
In addition to these examples, implementing typeof opens up countless possibilities for enhancing code flexibility and reliability while reducing potential errors. Whether it’s validating inputs, handling dynamic responses from APIs, or making conditional decisions based on types – the power of typeof truly shines in real-world scenarios.
By leveraging this feature effectively, developers can write more robust and maintainable code that adapts gracefully to various situations without sacrificing performance or readability. So next time you find yourself facing a complex problem requiring runtime type checking in TypeScript – don’t forget about the versatility offered by implementing typeof!
Remember: understanding how to implement typedef correctly ensures smoother development workflows while promoting cleaner codebases overall
Pros and Cons of using typescript typeof
The typeof operator in TypeScript can be a powerful tool for developers, but like any feature, it has its pros and cons. Let’s explore some of the advantages and disadvantages of using typeof.
One of the main benefits of using typeof is that it allows you to perform type checks at runtime. This can be especially useful when dealing with dynamic data or when working with third-party libraries that don’t have strong type definitions. The ability to check the type of a variable before executing code can help prevent runtime errors and improve overall program stability.
Another advantage is that typeof provides a way to handle cases where the actual type may vary. For example, if you’re expecting a variable to be either a string or an array, you can use typeof to determine which operations are valid for that variable at runtime. This flexibility can make your code more robust and adaptable.
On the flip side, one potential drawback of using typeof is that it relies on JavaScript’s built-in types rather than TypeScript’s more specific types. While this may not be an issue in many cases, there are situations where the information provided by typeof might not align perfectly with your desired TypeScript types.
Additionally, relying too heavily on typeof checks can lead to less readable code. When used excessively, these checks can clutter up your codebase and make it harder for other developers (or even yourself) to understand what’s going on.
Conclusion
In this comprehensive guide, we have explored the TypeScript typeof operator and its significance in TypeScript development. We began by understanding the basics of typeof and how it can be used to determine the type of a variable or expression at runtime.
We then delved into why typeof is important in TypeScript, highlighting its role in enabling developers to write more robust and error-free code. By providing type information, typeof helps catch potential bugs during compile-time rather than waiting for them to occur at runtime.
Next, we examined the basic syntax and usage of typeof, covering examples that demonstrated how it can be employed with different types such as string, number, boolean, arrays, functions, classes, and more. This knowledge will empower you to leverage typeof effectively in your own projects.
Additionally, we addressed common misconceptions about typeof that may lead to confusion among developers. By clarifying these misunderstandings surrounding null values or complex types like objects or interfaces when using typeof, you will have a clearer understanding of how to apply it correctly.
Furthermore, we explored advanced usage scenarios where typeof shines. We discussed implementing conditional types with keyof and mapped types using ReturnType. These techniques demonstrate the versatility of using typeof beyond simple type checking.
To bring everything together and showcase real-world applications of typedefs utility function across various industries; from web development frameworks like Angular.js 2+ framework which leverages typedef keyword extensively through their DI system’s dependency injection decorators (e.g., @Injectable) all way down into both front-end libraries such as React Redux store API through middleware design pattern called “thunks” wrapper around async actions allowing side-effects handling asynchronously without violating functional programming principles nor sacrificing productivity gains achieved via static typing inherent within TypeScripts ecosystem!
Overall,
the TypeScript `typeof` operator is an invaluable tool for any developer working with TypeScript codebases.
By providing insight into variable types at compile-time,
it empowers us to write safer code
and catch potential errors early on.
Whether you’re a beginner or an experienced developer,
understanding