Introduction to ReactJS
Welcome to the exciting world of ReactJS! If you’re a web developer, chances are you’ve heard about this powerful JavaScript library taking the front-end development scene by storm. And if you haven’t, well, hold on tight because we’re about to embark on an unforgettable journey into its core – Class Components!
In this comprehensive guide, we’ll unravel the mysteries behind class components in ReactJS and explore why they play such a vital role in building dynamic and interactive user interfaces. Whether starting with React or looking to level up your skills, understanding class components is essential for mastering this popular framework.
So get ready as we dive deep into the world of class components in ReactJS and uncover their secrets one piece at a time. Don’t worry if you’re new to all of this – we’ll break it down step by step so that even beginners can grasp these concepts effortlessly.
Are you excited? Great! Let’s begin our quest into the fascinating realm of class components and discover how they can supercharge your web development projects like never before!
Class Components vs Functional Components
ReactJS is a popular JavaScript library that allows developers to build user interfaces efficiently. When working with React, you have the option to use either class components or functional components. But what are the differences between these two?
Functional components are simpler and easier to understand compared to class components. They are JavaScript functions that return JSX code. You can think of them as pure functions, as they take in props and return elements without managing any state.
On the other hand, class components are more complex but offer additional functionality. They are implemented using JavaScript classes and have a special method called render(), which returns JSX code. Class components also have access to lifecycle methods like componentDidMount() and componentDidUpdate(), making it easier to manage component states.
So when should you use each type? It’s generally recommended to use functional components whenever possible due to their simplicity and performance advantages. If your component doesn’t need state management or lifecycle methods, then a functional component is sufficient.
However, if your component requires state management or access to lifecycle methods for tasks like fetching data from an API or setting intervals, then a class component would be more suitable.
In recent years, there has been a shift towards using functional components with the introduction of React hooks, which allow you to add stateful logic into functional components without converting them into classes.
Choosing the class or functional components depends on your project requirements and personal preference. Both options have their strengths and weaknesses; it’s up to you as a developer to decide which approach suits your needs best.
The Basics of Class Components
In ReactJS, components are the building blocks of any application. They allow you to create reusable and modular pieces of code that can be combined to form complex user interfaces. There are two types of components in React: functional components and class components.
Class components are an essential part of React development. They are written as ES6 classes and extend the base Component class provided by the React library. This allows them to have more features and functionality compared to functional components.
To create a class component, you simply define a new class that extends from React’s Component class. Inside this class, you define your component’s render method, which returns the JSX (JavaScript XML) that describes what should be rendered on the screen.
One key feature of class components is their ability to manage state. The state represents the data that changes over time in your application. With a simple syntax, you can initialize the state within your constructor method and update it using setState whenever necessary.
Props are another important concept in class components. Props allow you to pass data from a parent component down to its child component, making it easy to share information between different parts of your application.
Lifecycle methods play a crucial role in managing the lifecycle events or phases of a component – from initialization, rendering, and updating until unmounting. These methods give you control over when certain actions should occur during these phases.
Some commonly used lifecycle methods include componentDidMount(), componentDidUpdate(), componentWillUnmount(), etc., where you can perform tasks like fetching data from an API or cleaning up resources before your component is removed from the DOM.
Understanding how these basics work together will set a solid foundation for building powerful applications with ReactJS using class components.
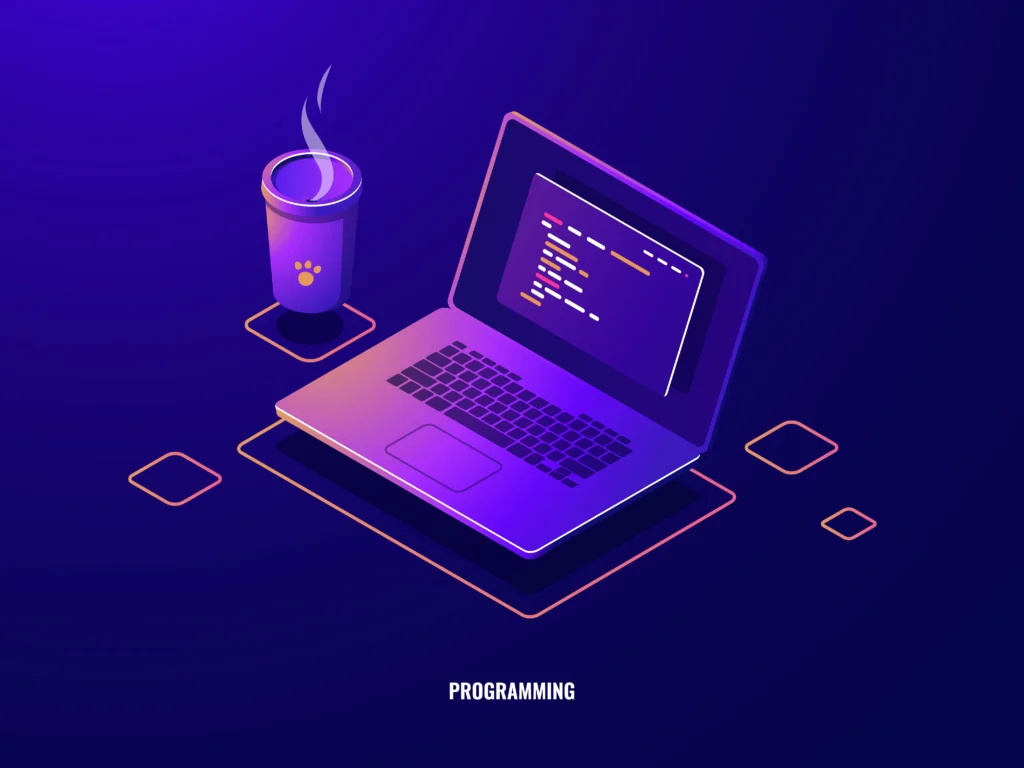
State and Props in Class Components
One of the fundamental concepts to understand when working with class components in ReactJS is the concept of state and props. State refers to the internal data of a component, while props are used for passing data from a parent component to its child components.
In class components, the state is declared using the constructor method. It allows you to initialize and manage the state object within your component. The state can be updated by calling this.setState(), which triggers a re-rendering of the component.
Props, on the other hand, are passed down from parent components to child components as immutable properties. They provide a way for different parts of your application to communicate with each other. To access props within a class component, use this.props.propertyName.
The key difference between state and props is that state is managed internally within a component, while props are passed down from parent components. State represents data that can change over time based on user interactions or external factors. In contrast, props remain constant throughout the lifetime of a component unless the parent explicitly changes them.
Understanding how to handle both state and props properly is crucial for building complex applications with ReactJS using class components. By effectively managing these two concepts, you can create interactive and dynamic UIs that respond seamlessly to user input.
Stay tuned as we delve further into exploring lifecycle methods in class components!
Lifecycle Methods in Class Components
Class components in ReactJS have a set of built-in methods, known as lifecycle methods, that allow you to control different stages of a component’s life cycle. These methods are triggered at specific points during the rendering and re-rendering process.
One commonly used lifecycle method is componentDidMount(). This method is called immediately after the component has been rendered on the screen. It is often used for initializing data or making API calls.
Another important lifecycle method is componentDidUpdate(). This method is called whenever there are updates to the component’s props or state. It can be useful for performing actions based on these changes, such as updating data or triggering animations.
The component will unmount () method is called right before the component gets removed from the DOM. It provides an opportunity to clean up any resources the component allocates, such as event listeners or timers.
There are several other lifecycle methods available, each serving a specific purpose in managing your components’ behaviour. For example, shouldComponentUpdate() allows you to optimize performance by preventing unnecessary re-renders based on certain conditions.
Understanding and utilizing these lifecycle methods effectively can greatly enhance your ability to control and manage your ReactJS class components throughout their lifespan.
Remember that with the introduction of React Hooks in newer versions of React, functional components offer alternative solutions for handling component lifecycles without relying solely on class components. However, if you’re working with an older codebase or prefer using class-based syntax, mastering these lifecycle methods remains crucial.
Mastering the various lifecycle methods available in class components gives you fine-grained control over how your components behave at different stages of their existence within a ReactJS application. Whether fetching initial data when mounted or cleaning up resources when unmounted – understanding and utilizing these techniques will help ensure smooth functioning and efficient app rendering.
Building a Simple React App with Class Components
Now that we have a good understanding of class components in ReactJS let’s put our knowledge into practice by building a simple React app. This will give us a hands-on experience and help solidify our understanding of class components.
First, let’s start by creating the basic structure of our app. We must set up the necessary files and folders, such as index.js and App.js. Inside the App.js file, we can begin by importing React and extending the Component class.
Next, we can create our constructor method within the class component. Here is where we can initialize state variables for our app. The state allows us to store and manage data that may change over time.
Once we have set up our constructor method, it’s time to render some content onto the screen. We can implement the render() method within our class component. This is where we return JSX elements that make up the user interface of our app.
To add interactivity to our app, we can define methods within our class component that handle specific actions or events triggered by user input or other factors. These methods can be called from within JSX elements using event handlers like onClick or onSubmit.
As you build your simple React app with class components, remember lifecycle methods! These methods allow you to perform certain actions at different stages of a component’s life cycle – when it mounts or unmounts, updates occur, etc.
Remember always to keep your code organized and modularize your components whenever possible. This makes it easier to understand and maintain your codebase as your application grows.
With these steps in mind, you should now have a strong foundation for building simple React apps using class components in ReactJS!
Stay tuned for more advanced topics on ReactJS coming soon!
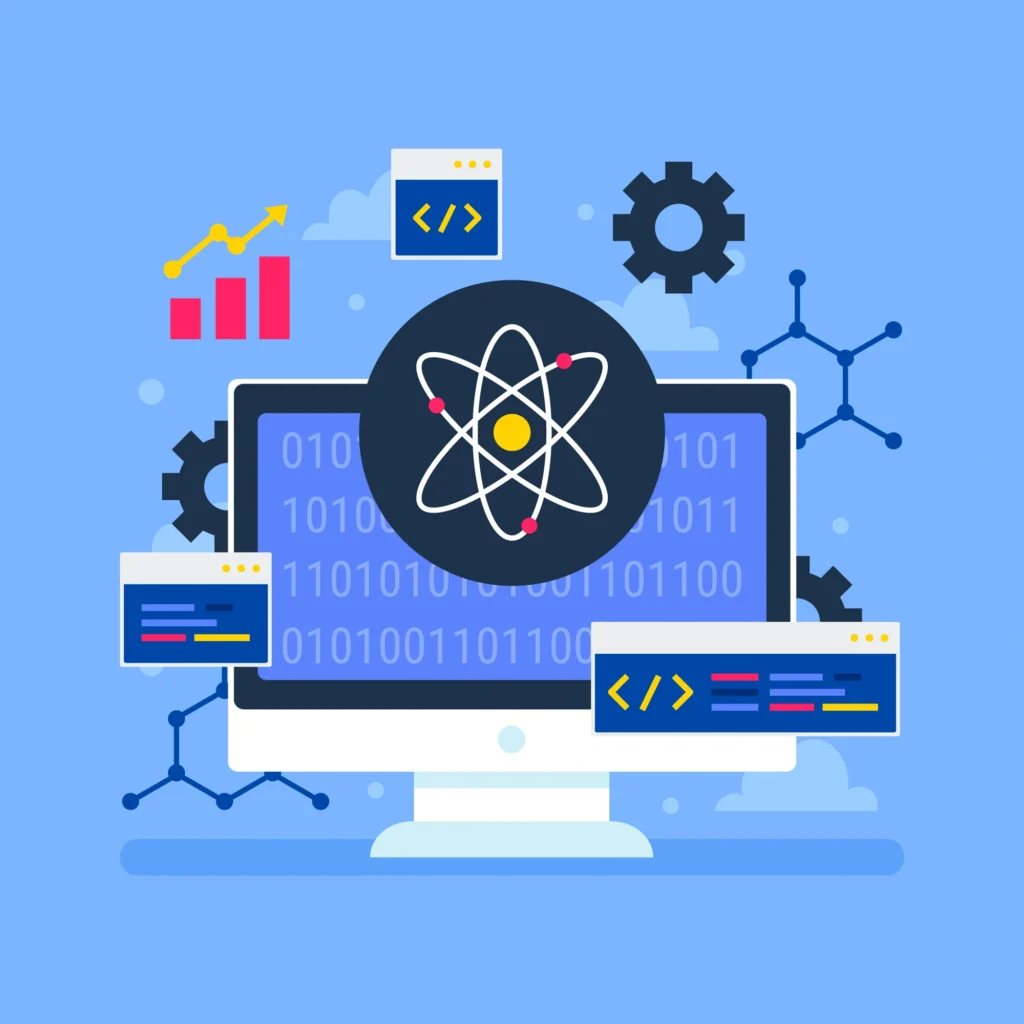
Best Practices for Using Class Components
When working with class components in ReactJS, some best practices can help improve the readability and maintainability of your code.
It’s important to keep your class components focused and modular. Each component should have a single responsibility and be kept as small as possible. This makes it easier to understand and debug your code.
Another best practice is to use concise naming conventions for your class methods. By giving them descriptive names that accurately reflect their purpose, you make it easier for other developers (and yourself) to understand what each method does at a glance.
Additionally, avoid directly mutating the state object within your class components. Instead, use the `setState` method provided by React to update the state in an immutable way. This ensures that the component properly tracks any changes made to the state and triggers a re-rendering when necessary.
It’s also recommended to leverage lifecycle methods effectively. Understand how they work and utilize them appropriately to perform tasks such as fetching data from APIs or cleaning up resources before a component is unmounted.
Consider using functional components instead of class components whenever possible. Functional components offer better performance due to their lightweight nature and encourage writing more reusable code through hooks like useState and useEffect.
By following these best practices, you can write clean, efficient, and maintainable class components in ReactJS that will enhance both the development process and user experience.
Conclusion
In this comprehensive guide, we have delved into the world of class components in ReactJS. We started by understanding the basics of ReactJS and then explored the differences between class and functional components. After That We learned that while functional components are simpler and more lightweight, class components offer additional features such as state and lifecycle methods.
We then dived deeper into the workings of class components, exploring how to use state and props to manage data within our applications. By leveraging these powerful concepts, we can create dynamic and interactive user interfaces.
Next, we explored the lifecycle methods available in class components. These methods allow us to control what happens at different stages of a component’s life cycle, giving us greater flexibility in managing our application’s behaviour.
To put our newfound knowledge into practice, we built a simple React app using class components. This hands-on experience helped solidify our understanding of how these building blocks come together to create dynamic web applications.
We discussed some best practices for using class components effectively. By following these guidelines, we can write cleaner code that is easier to maintain and understand.
As you continue your journey with ReactJS development, it is important to remember that both functional components and class components have their own merits. While functional components are gaining popularity due to their simplicity and performance benefits, there may still be situations where using a class component is necessary or advantageous.